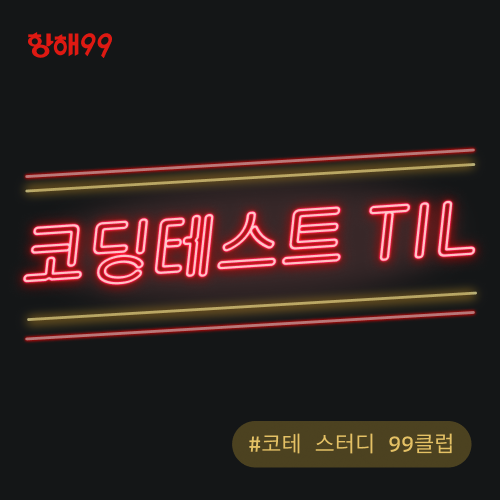
✨ 문제
Decode the Message
You are given the strings key and message, which represent a cipher key and a secret message, respectively. The steps to decode message are as follows:
- Use the first appearance of all 26 lowercase English letters in key as the order of the substitution table.
- Align the substitution table with the regular English alphabet.
- Each letter in message is then substituted using the table
- Spaces ' ' are transformed to themselves.
- For example, given key = "happy boy" (actual key would have at least one instance of each letter in the alphabet), we have the partial substitution table of ('h' -> 'a', 'a' -> 'b', 'p' -> 'c', 'y' -> 'd', 'b' -> 'e', 'o' -> 'f').
https://leetcode.com/problems/decode-the-message/description/
✨ 개념
해시 테이블 Hash Table
- 해시 테이블은 키-값 쌍을 저장하는 자료구조
- 키를 해시 함수에 전달하여 해시 코드를 생성하고, 이 해시 코드를 배열의 인덱스로 사용해 데이터 저장/검색
- 동기화된 컬렉션으로, 여러 스레드가 동시 접근해도 안전함.
✨ 최종코드
class Solution {
public String decodeMessage(String key, String message) {
Hashtable<Character, Character> hashTable = new Hashtable<Character, Character>();
char[] answer = new char[message.length()];
// 공백 제거
String replaceKey = key.replace(" ", "");
char chr = 'a';
for (char c : replaceKey.toCharArray()){
if (!hashTable.containsValue(c))
hashTable.put(chr++, c);
}
int i = 0;
for (char c : message.toCharArray()) {
if (c == ' ') {
answer[i++] = c;
continue;
}
for (Map.Entry<Character, Character> entry : hashTable.entrySet()) {
if (entry.getValue().equals(c)) {
answer[i++] = entry.getKey();
break;
}
}
}
return new String(answer);
}
}
Topic을 보니 HashTable을 사용해야하는 것 같아서 써봤더니 코드가 굉장히 지저분해졌다. 깔끔하게 보완하고 싶지만 너무 졸리다.
일단 hashtable을 생성하여 매개변수로 받은 key를 키값을 순서대로 a부터 넣어주었다. 그리고 message를 디코딩하기 위해 entrySet을 만들어 value값으로 key값을 받아오도록 하였다. key는 공백을 제거해야하고 message는 공백을 그대로 출력해야해서 구현하다보니 지저분해졌다. 추후에 수정해보도록 하겠다.
'알고리즘 > 99클럽' 카테고리의 다른 글
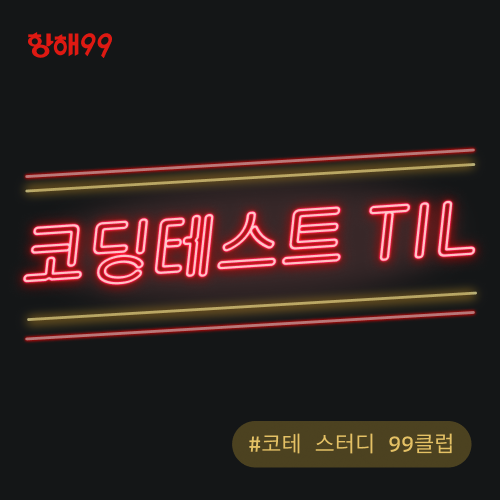
✨ 문제
Decode the Message
You are given the strings key and message, which represent a cipher key and a secret message, respectively. The steps to decode message are as follows:
- Use the first appearance of all 26 lowercase English letters in key as the order of the substitution table.
- Align the substitution table with the regular English alphabet.
- Each letter in message is then substituted using the table
- Spaces ' ' are transformed to themselves.
- For example, given key = "happy boy" (actual key would have at least one instance of each letter in the alphabet), we have the partial substitution table of ('h' -> 'a', 'a' -> 'b', 'p' -> 'c', 'y' -> 'd', 'b' -> 'e', 'o' -> 'f').
https://leetcode.com/problems/decode-the-message/description/
✨ 개념
해시 테이블 Hash Table
- 해시 테이블은 키-값 쌍을 저장하는 자료구조
- 키를 해시 함수에 전달하여 해시 코드를 생성하고, 이 해시 코드를 배열의 인덱스로 사용해 데이터 저장/검색
- 동기화된 컬렉션으로, 여러 스레드가 동시 접근해도 안전함.
✨ 최종코드
class Solution { public String decodeMessage(String key, String message) { Hashtable<Character, Character> hashTable = new Hashtable<Character, Character>(); char[] answer = new char[message.length()]; // 공백 제거 String replaceKey = key.replace(" ", ""); char chr = 'a'; for (char c : replaceKey.toCharArray()){ if (!hashTable.containsValue(c)) hashTable.put(chr++, c); } int i = 0; for (char c : message.toCharArray()) { if (c == ' ') { answer[i++] = c; continue; } for (Map.Entry<Character, Character> entry : hashTable.entrySet()) { if (entry.getValue().equals(c)) { answer[i++] = entry.getKey(); break; } } } return new String(answer); } }
Topic을 보니 HashTable을 사용해야하는 것 같아서 써봤더니 코드가 굉장히 지저분해졌다. 깔끔하게 보완하고 싶지만 너무 졸리다.
일단 hashtable을 생성하여 매개변수로 받은 key를 키값을 순서대로 a부터 넣어주었다. 그리고 message를 디코딩하기 위해 entrySet을 만들어 value값으로 key값을 받아오도록 하였다. key는 공백을 제거해야하고 message는 공백을 그대로 출력해야해서 구현하다보니 지저분해졌다. 추후에 수정해보도록 하겠다.